A Completely Platform-Independent Interface
- wu iris
- Sep 16, 2020
- 3 min read
Downloadable
Controls:
Exit: ESC or click X
Pause/Resume: Space
Key Points
The main ideas of Assignment 3 are to eliminate all differences between Graphics.d3d.cpp and Graphics.GL.cpp and then initialize Mesh/Effect with data so that we can create multiple meshes or effects easily.
In my opinion, the most important questions are as follows:
Eliminate Differences
It was pretty clear that last week's assignment didn't solve every difference between Graphics.d3d.cpp and Graphics.GL.cpp, especially the d3d platform, since it needs to initialize views before it initializes any other things. But that made me think why GL didn't need that function so I took a look at what the i_initializationParameters are. Apparently, the gl platform gets an instance of the application while the d3d platform gets the width and height, which is not "fair" in my opinion. What's more, initializing views should be a prerequisite so it's a little weird to put the definition in Graphics.cpp. Therefore I decided to put it into Context, since it's not like Mesh or Effect that we may want to have multiple instances of them. We only need one. I also put the rest of the rendering code in Context and created two interfaces (one for clearing screen, one for displaying), since both of them are called only once per frame and are applied to the whole game. Implementations were put into sContext.d3d.cpp and sContect.gl.cpp.
After refactoring, the code that clears the back buffer became very simple:
float background[3] = { 0.0f, 0.0f, 0.0f };
sContext::g_context.ClearScreen(background);
(Alpha is a separate parameter and is set to 1.0f by default.)
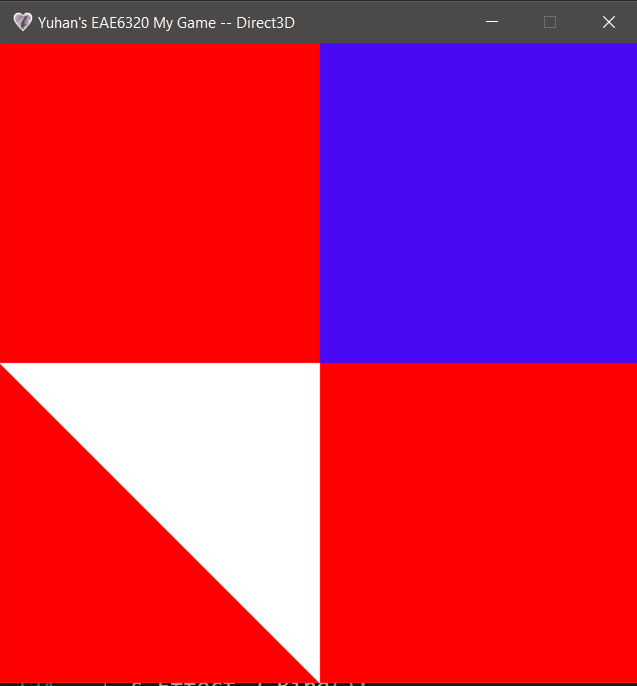
From my experience in making the game pause, I used constantData_frame.g_elapsedSecondCount_simulationTime to get simulation time. Similar to assignment 1, cos and sin were used to make the background color animate.
New Initialization Interface
I’ve already rewrote the initialization interface of Effect class last time, because it was pretty clear that all it needed were two files:
s_Effect_1.InitializeShadingData(vertex_path, fragment_path)
Effect takes up 48 bytes in d3d while it’s only 16 bytes in gl. Here are some variables from cEffect.h:
cShader* m_VertexShader = nullptr;
cShader* m_FragmentShader = nullptr;
cRenderState m_RenderState;
#if defined( EAE6320_PLATFORM_GL )
GLuint m_ProgramId = 0;
At first, I was surprised, since there seemed to be more variables in gl (if we don't look at cRenderState). Then I remembered one was 64 bits and one was 32 bits, so the sizes of pointers were different. To make it smaller, I think we can probably use a pointer for cRenderState. I know this works since I once tried it. However, sometimes I just avoid using pointers if it doesn't improve much. After all, we tend to make more mistakes when it comes to pointers.
I was pretty confused about the initialization interface of Mesh when I was doing assignment 2, but after I learned how to draw with indices, with abstraction, things became clearer. First of all, TriangleCount and VertexPerTriangle are used for both initialization and rendering, so it’s something that needs to be stored. But vertex data and index data are not. They are put into a buffer during initialization and we don’t need that for rendering. After refactoring, the function call is:
s_Mesh_1.InitializeGeometry(shapeCount_1, vertexCountPerShape, vertexData_1, indexData_1)
To convert indices from left-handed to right-handed, I reverse every three elements in the index array.
Data is as follows:
unsigned int m_ShapeCount = 2;
unsigned int m_VertexCountPerShape = 3;
#if defined( EAE6320_PLATFORM_D3D )
cVertexFormat* m_VertexFormat = nullptr;
ID3D11Buffer* m_VertexBuffer = nullptr;
ID3D11Buffer* m_IndexBuffer = nullptr;
#elif defined( EAE6320_PLATFORM_GL )
GLuint m_VertexBufferId = 0;
GLuint m_VertexArrayId = 0;
GLuint m_IndexBufferId = 0;
Mesh takes up 32 bytes in d3d while it’s 20 bytes in gl. Again, pointers in d3d are 64 bits, so apparently, it should take up more space. I feel this is probably the smallest size I can get, since everything is used in multiple places.
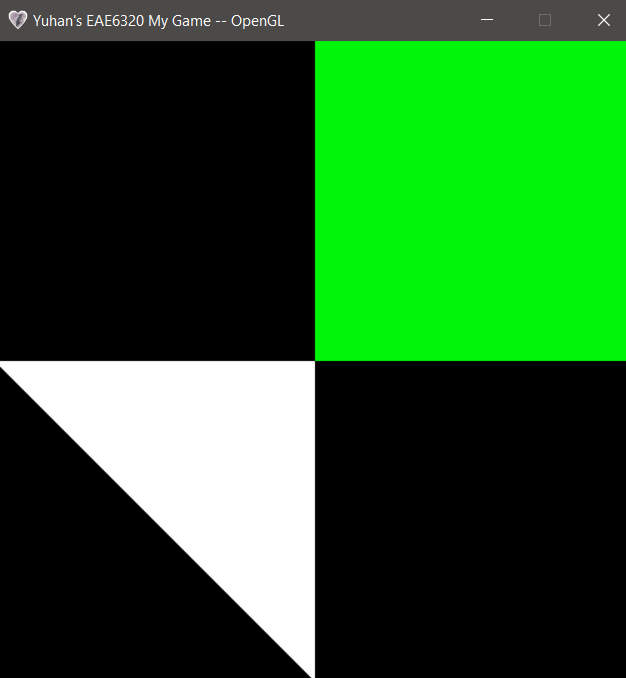
Comments