Threading
- wu iris
- Sep 22, 2020
- 3 min read
Downloadable
Controls:
Exit: ESC or click X
Pause/Resume: Space (hold)
Hide Mesh: Shift (hold)
Change Effect: Ctrl (hold)
Key Points
Assignment 4 is to use threads so that while the graphics library is rendering the current frame, our game can still submit the data to be rendered in the next frame.
Count Reference
Reference counting is very important when it comes to threading, since our game could possibly release the object before the graphics library renders it. The method used in this assignment is using a variable to store the number of “owners” of this object, which works, however, if we forget to increment/decrement, it can cause great problems(e.g. memory is not released). That’s because it is not controlled by the class itself or done “automatically”, so we always need to remember to increment/decrement. Smart pointer is definitely a better choice, though we might need to take a lot of operations into consideration.
After my first refactoring, the Mesh was 40 bytes in D3D and 24 in GL, while the Effect was 56 in D3D and 20 in GL. Compared with data from last week, apparently, more than 2 bytes (the size of uint16_t) were added to the size of the class. But the difference drew my attention and I started thinking about whether it was due to alignment. That was when I realized I might not have gained a smaller size last week because two new variables were unsigned int instead of a smaller type. After changing types and moving smaller types to the end, the Mesh is now 32 bytes in D3D and 20 in GL, while the Effect is still 56 in D3D and 20 in GL. The size of mesh significantly decreased in D3D not only because of type change, since int takes up 4 bytes on both platforms. Better arrangement also made those variables take up less space.


Make Mesh/Effect Change
To change Mesh/Effect change while the game is still running, I override the virtual function UpdateSimulationBasedOnInput() and UpdateSimulationBasedOnTime(const float i_elapsedSecondCount_sinceLastUpdate) in iApplication. Similar to how exiting and pausing works, the first function checks player input and stores states in boolean variables, so that later when submitting data, I can check whether these variables are set or not. The second function is used to change Mesh/Effect automatically as time goes by. I used another variable to store the time that has passed so that I know when I should make changes.
Submit Data
I use an array of 4 floating numbers as the parameter to submit background color:
float background[4] = { choice1, choice2, choice1, 1.0f };
eae6320::Graphics::SubmitBackgroundColor(background);
To make it animate, I call the function named GetElapsedSecondCount_simulation() to get simulation time, and then, same as usual, use cos, sin, and abs to get a number between 0 and 1.
For Mesh/Effect pair, I chose a rather simple interface:
eae6320::Graphics::SubmitMeshEffectPair(submit_effects, submit_meshes, curIndex);
The first two parameters are arrays of effects and meshes. Each item in submit_effects maps to one item in submit_meshes. The third parameter indicates how many Mesh/Effect pairs that the graphics library needs to render in this frame. This is probably not the best way, but while I was working on this part, I was thinking that I didn't want to emphasize too much on the idea of "pair" since when one effect is bound, it can be applied to all meshes unless I switch to another one. To avoid passing in too much information, I added an assertion only in the debug version(through macros), though, in the release version, it processes the maximum number of effects/meshes it can process. The reason why we are doing this is because rendering takes more time than deciding what we want to render and also these two actions can be separated from each other.


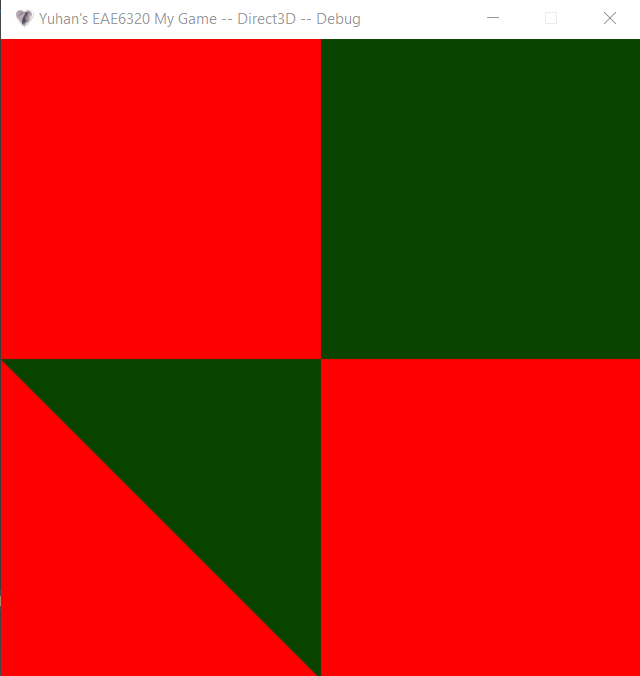
After changing the structure of sDataRequiredToRenderAFrame, each one now takes up 192 bytes in D3D and 176 bytes in GL, though sFrame already takes up 144 bytes. So the total, including the size of two buffers, should be 400 in D3D and 360 in GL. I don’t think the data that pointers are pointing at should be counted in, for the graphics library doesn’t need to know what these pointers are actually pointing at when rendering.
Comments